Build a CRUD App with a Firestore Database
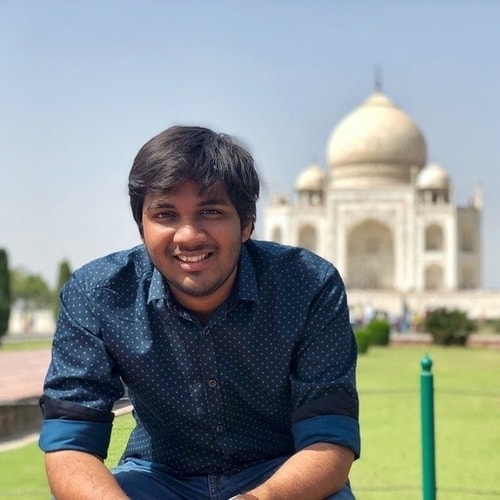
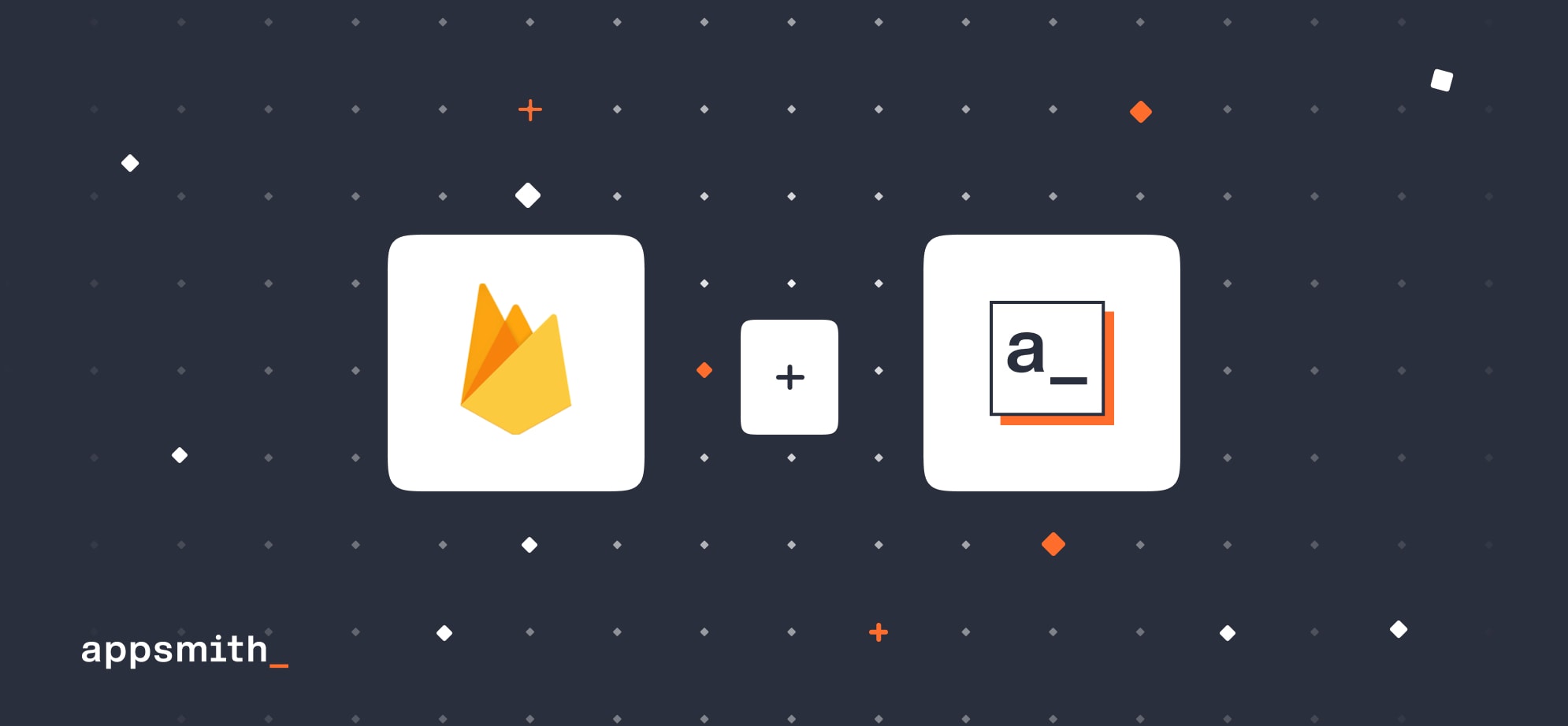
Low-code-based web applications are often discussed when talking about the future of software development. While some developers call it 'no-code,' others call it 'low code'; no matter what you want to call them, they are showing no signs of slowing down in growth among small to large businesses, especially when this technology is bringing about a great change even on the back-end side of things. One major technology that seems to have worked well in this market is Google's Firebase. It is a platform for app building created by Google. Firebase uses an open-source development framework making it very accessible for developers to swiftly prototype and integrate them into their apps. With this, Firebase and its database Firestore, most back-end needs are fulfilled without writing code. But you can't build web-based internal applications/admin panels with front-end frameworks simultaneously because building UI from scratch is not easy.
This part, however, can be simplified with Appsmith, where you can create a fully functional and custom front-end in minutes. A vast array of pre-built UI components, that is, widgets, are available to help you build good-looking applications. Connecting data sources with Appsmith takes only a few minutes, and you can quickly build tools on top of the database of your choice.
This blog will teach you how to build a front-end that can connect to Firestore as a datasource.
“”Firestore is a NoSQL document database built for automatic scaling, high performance, and ease of application development. While the Firestore interface has many of the same features as traditional databases, as a NoSQL database, it differs from describing relationships between data objects.
Getting Started: Connecting Firestore on Appsmith
On Appsmith, it's pretty straightforward to establish a connection with any datasource, including Firestore.
What we need to make the connection are the Database URL, Project Id, and Service Account Credentials. With this in mind, let's get started.
Create a new account on Appsmith (it's free!), if you are an existing user, log in to your Appsmith account.
Create a new application under the dashboard under your preferred organization.
On your Appsmith application, click on the + icon next to Datasources on the left navigation bar under Page1
Next, click on the Now, navigate to the Create New tab and choose Firestore datasource; you'll see the following screenshot:
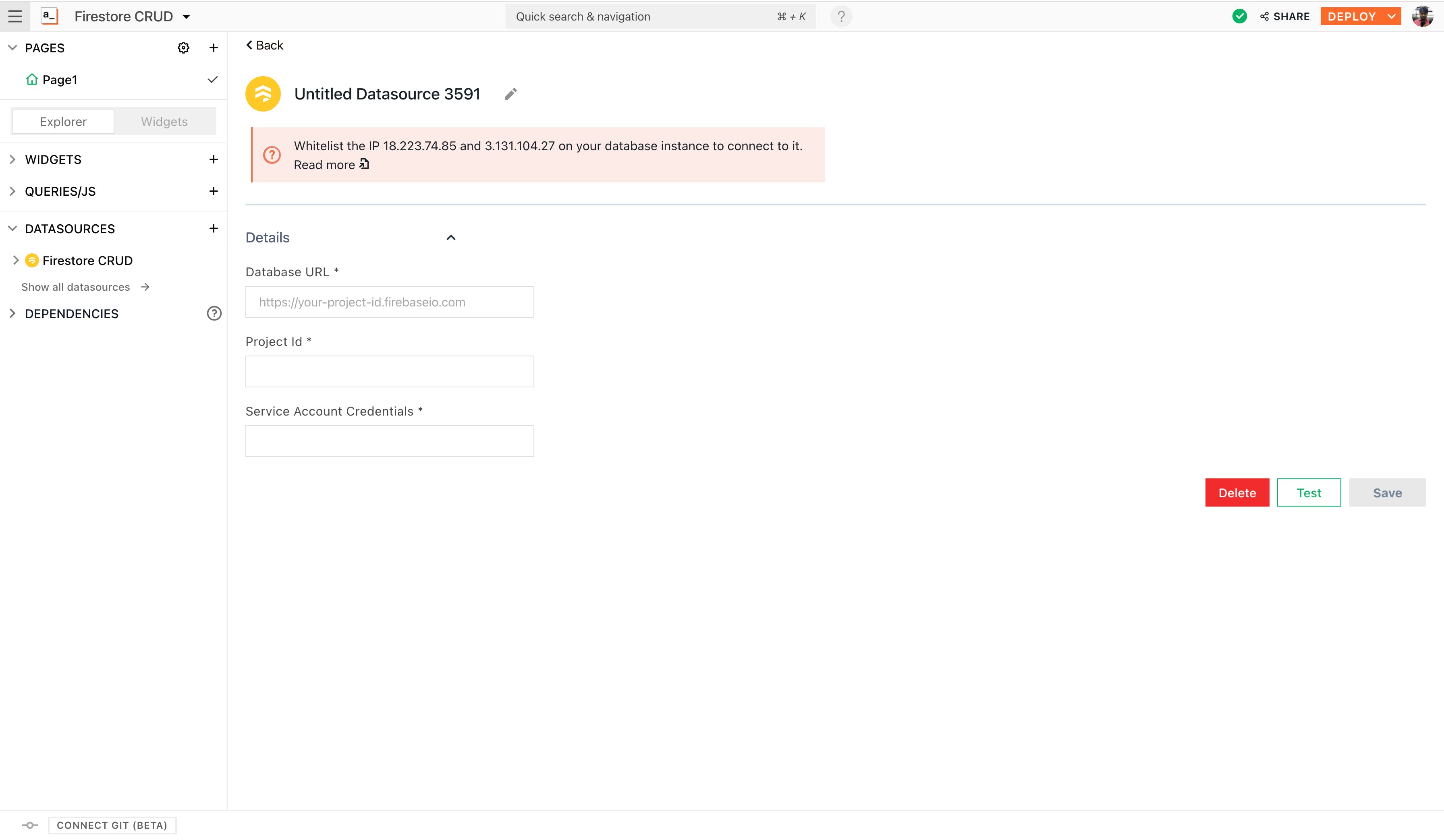
When using Firestore, all these details can be found under the firebase console under project settings.
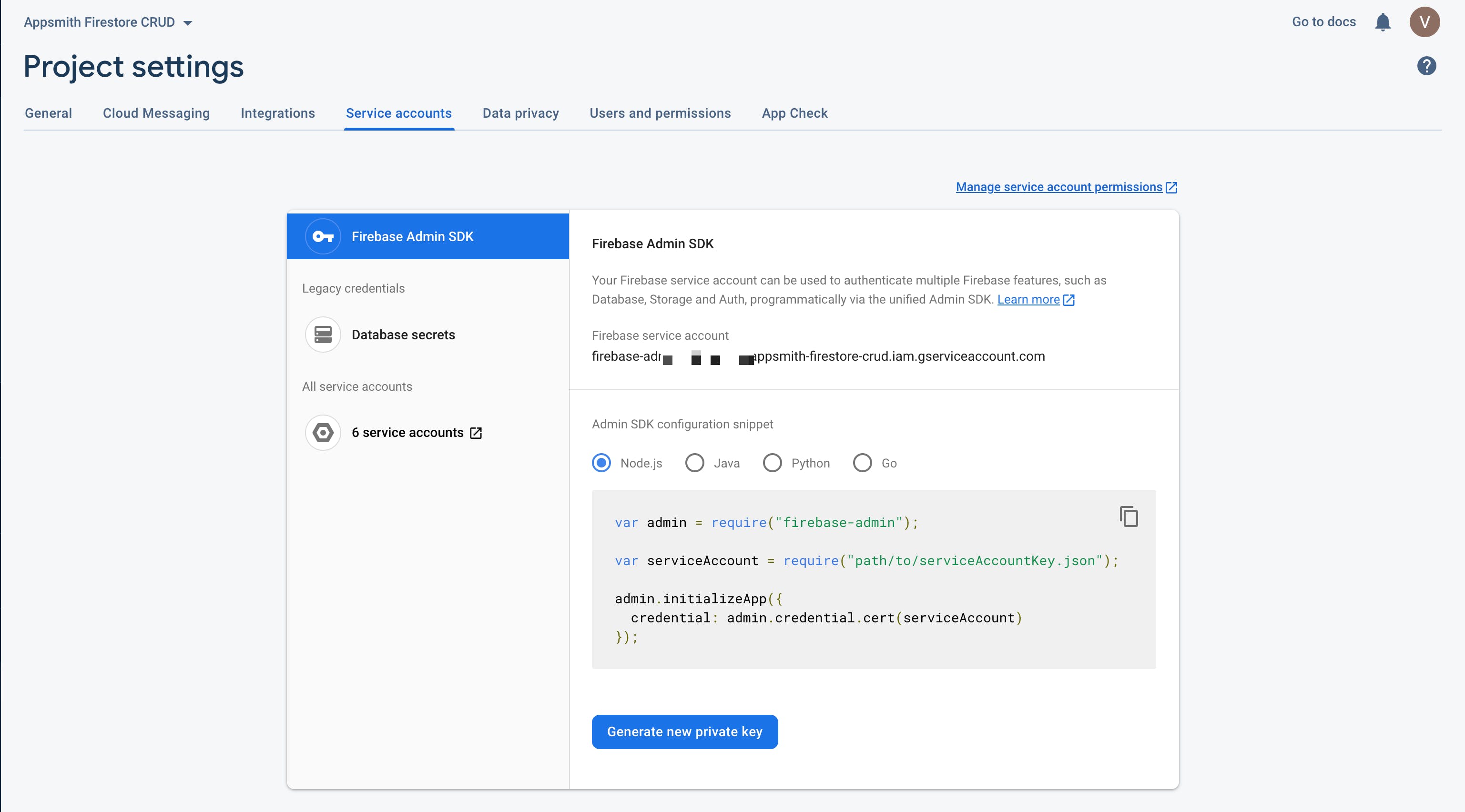
Rename the Datasource to Firestore CRUD by double-clicking on the existing one.
Here’s what the configuration would look like:
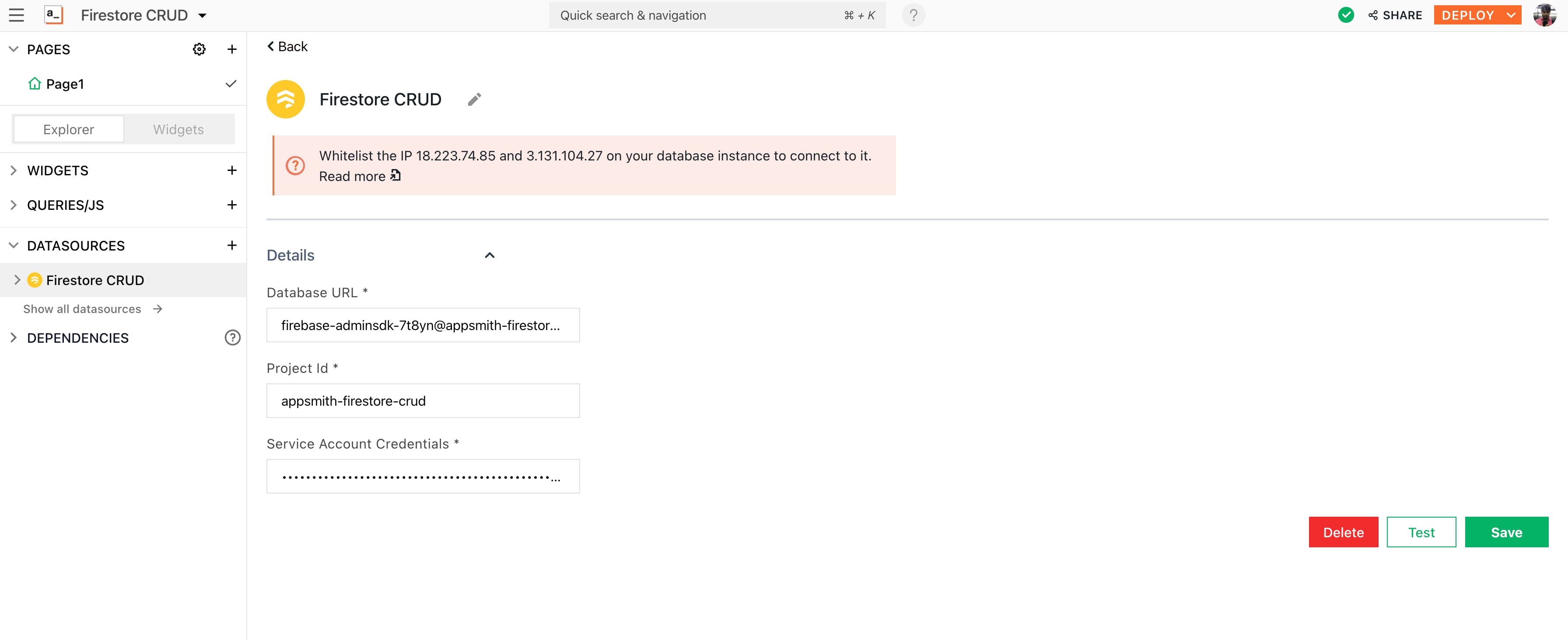
“”Note: For service account credentials, generate a new private key and copy its contents.
Next, click on the Test button at the bottom right of the screen. This will help you with understanding whether your configuration is valid or not. If it returns a successful message, hit the 'Save' button to establish a secure connection between Appsmith and Firestore.
Creating a new Table on Firestore
We are done with the basic configuration. Now, let's create a collection on Firestore to build a simple to-do list application and learn all the basic CRUD operations on top of Appsmith.
On Firestore it's super easy to do this from the console, just hit the create collection button on the dashboard, and define all the attributes in the model.
Followings are the attributes and data types we use:
name: string
assigned_to: string
complete: boolean
deadline: datetime
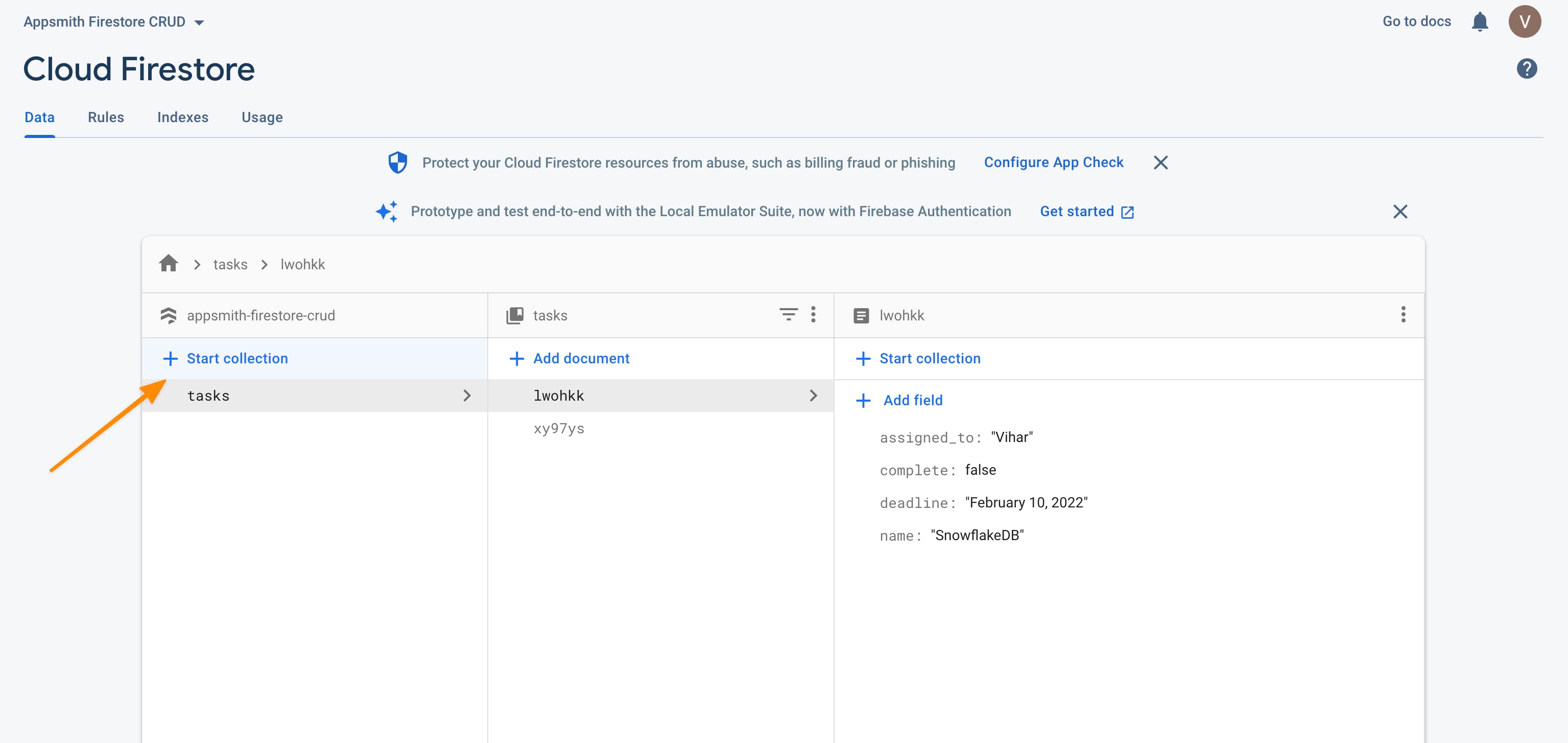
Alrighty, our collection is not created; let's get into CRUD.
CRUD on Firestore with Appsmith
Implementing the Read Operation
First, let's read our data from the database and display it on a beautiful table widget. Follow the below steps:
Click on the + icon next to the datasources and choose Create New + from the Firestore CRUD datasource.
Rename the query t getTasks
Set the query command to Get Documents in Collection
Set the Collection/Document Path * to collection name, which in our case is tasks
This simple query returns all the task details in the sample data item. Hit the RUN button to view all the results.
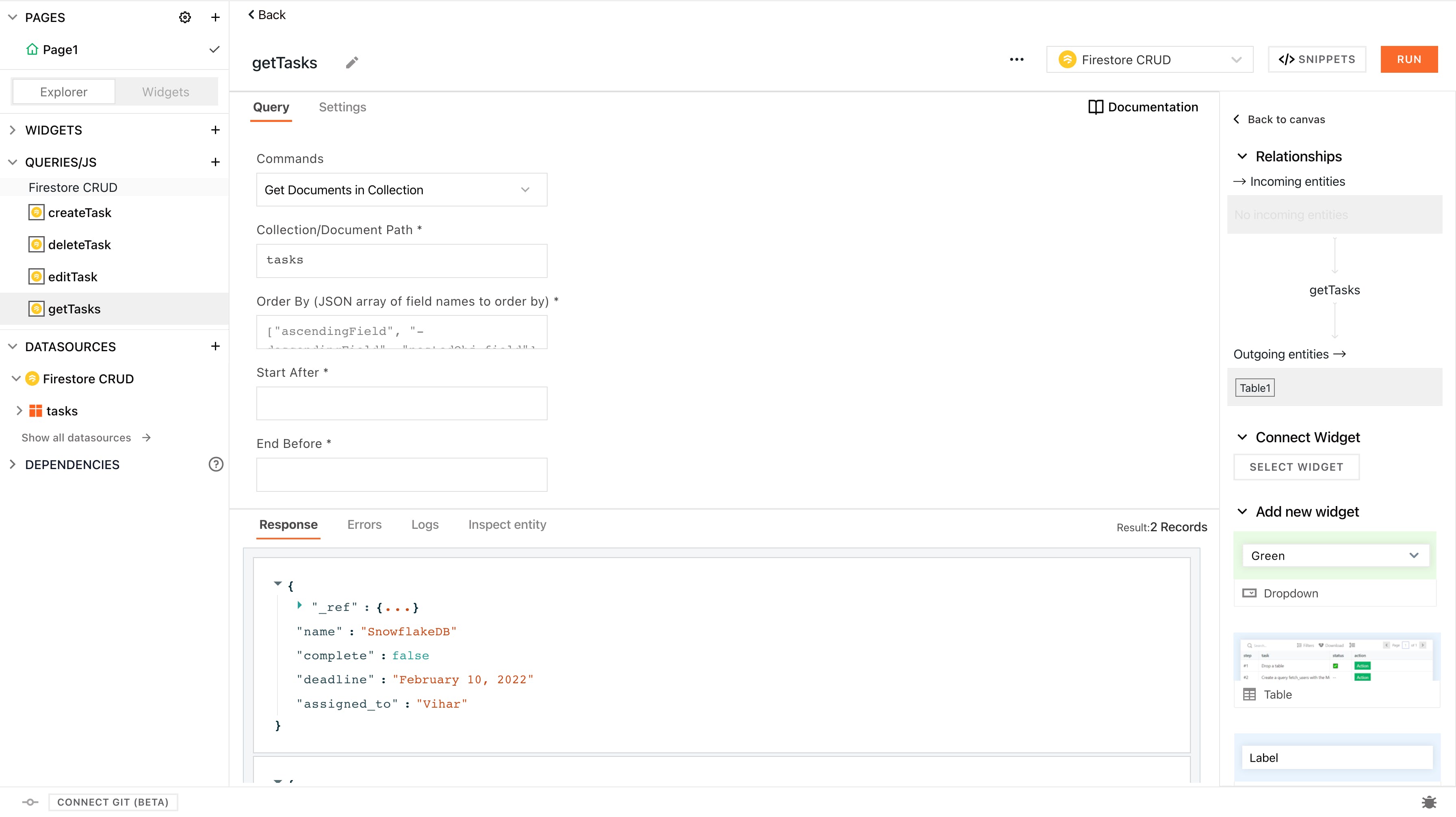
We now have our query; let's bind this onto the table widget; for this, follow the below steps:
Click the + icon next to widgets on the sidebar, search for the table widget, and drag and drop it onto the canvas.
You can make any configurations to any widget via the property pane. Click on the table widget on the canvas; you will see the property pane docked to the sidebar on the right. Now, under the Table Data property, use the moustache syntax to bind the query:
{{getTasks.data}}
With this, we should see all the data displayed on the table. The column names can be configured and re-organized under the property pane.
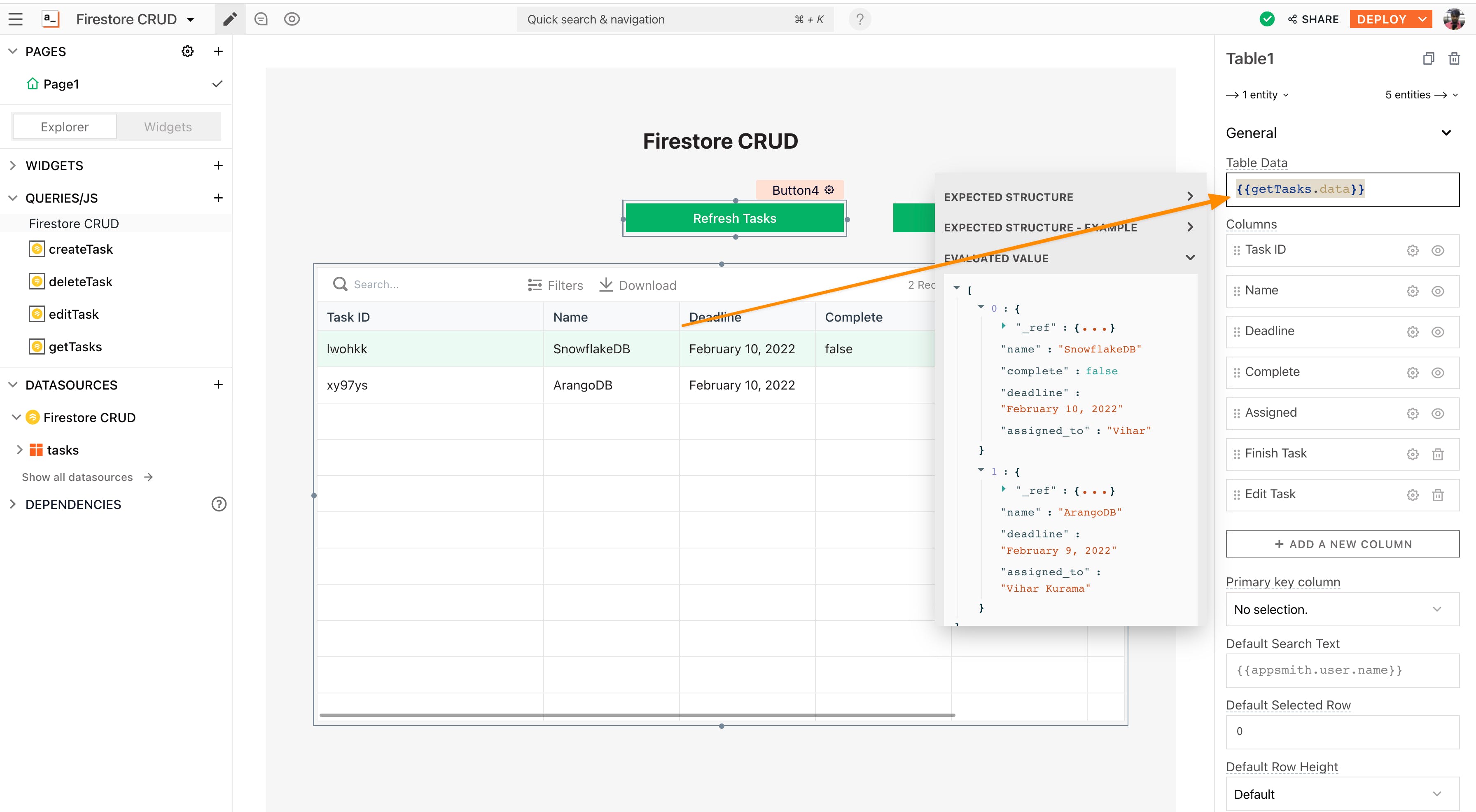
Implementing the Create Operation
To add the create operation on Firestore, let's make UI.
Drag and drop a button widget onto the canvas. Open its property pane, set the onClick property to Open a New Modal, and choose Create New.
This will open up a new modal now; let's drag and drop a few widgets to create a form that we can use to add a new task into our database.
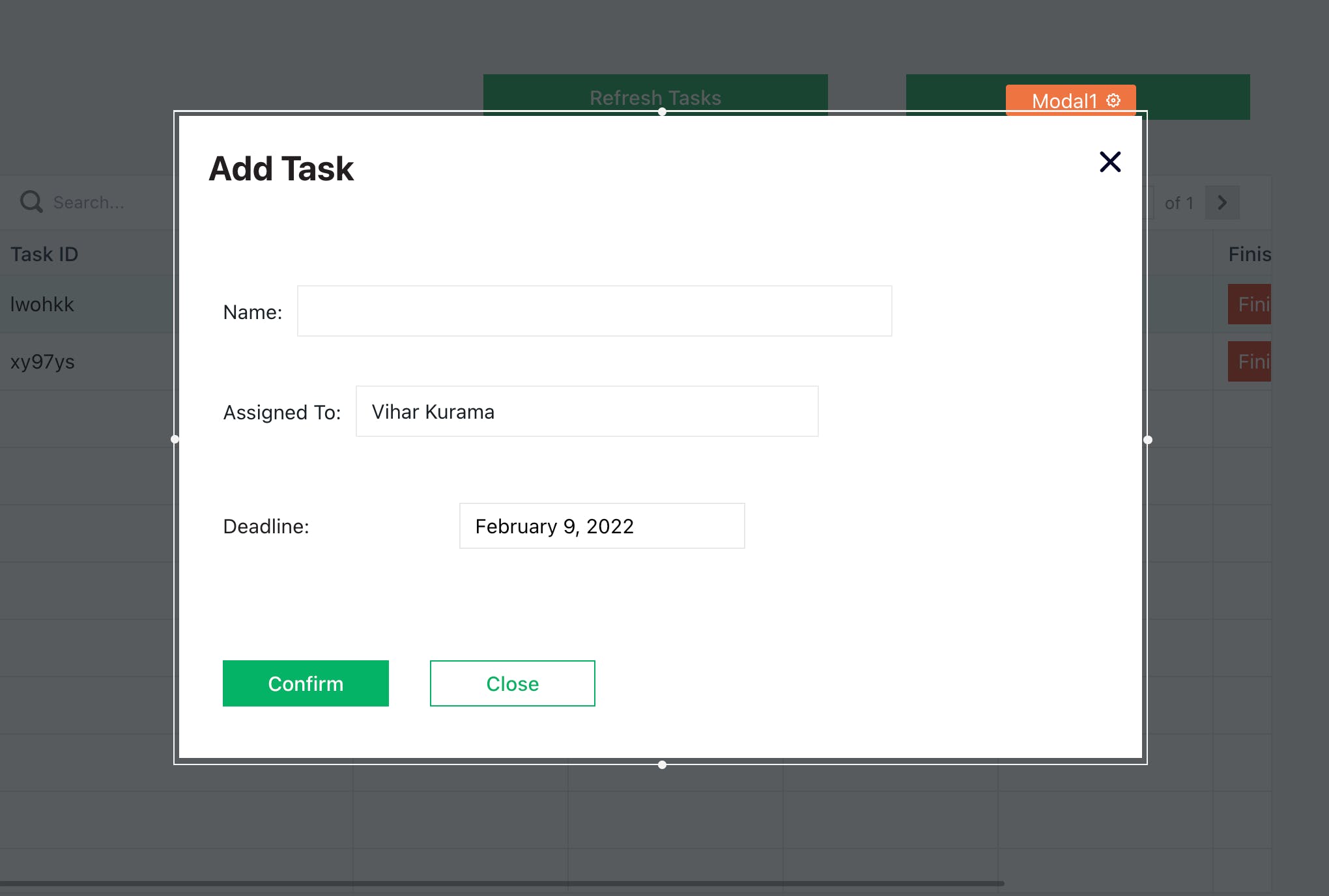
Here, we have three input widgets to add to our tasks. We can configure the default values, labels, and placeholders by selecting the respective property panes. Now, let’s write the query that lets us create a new task on Firestore.
Note: The default value is set to the logged-in user name using Appsmith’s context object, you can do this by binding {{appsmith.user.name}} in the default text property.
Follow the steps below:
Click on the + icon next to the datasources and choose to Create New + from the Firestore CRUD datasource
Rename the query to createTask
Set the commands to Create Document
Set the Collection/Document Path *to tasks/{{Math.random().toString(36).substring(7)}}
Finally, set the body property to:
{
"name":"{{Input1.text}}",
"deadline": "{{DatePicker1.formattedDate}}",
"assigned_to": "{{Input2.text}}",
"complete": false
}
Here, we have an insert query that collects all the data from the form widgets we've created. Note that we use the moustache syntax to bind the data from the widgets onto the query body.
Lastly, we’ll need to configure the submit button; for this, go back to the modal and set the button’s onClick property to execute a query and choose createTask under the events property:
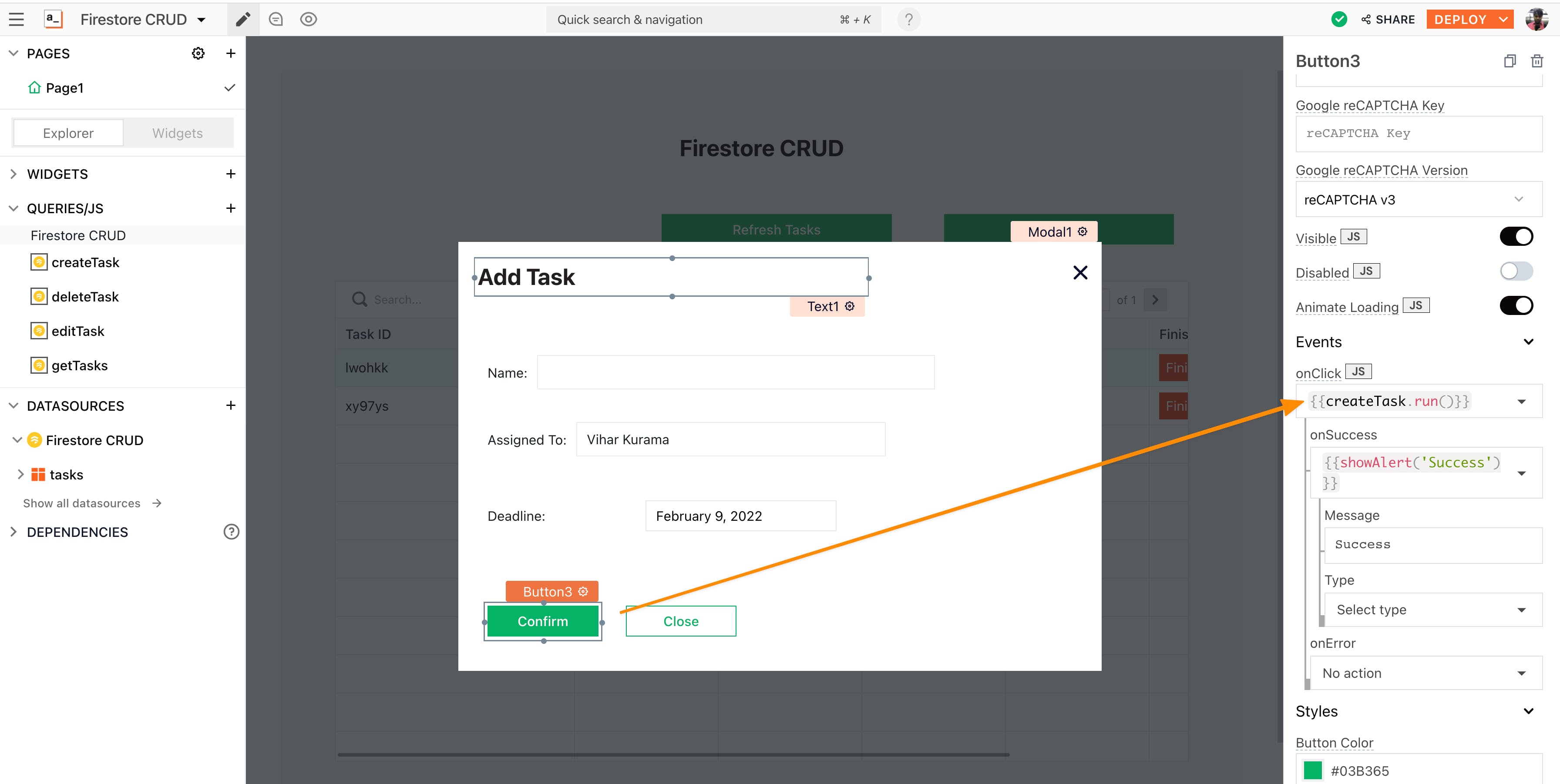
Implementing the Update Operation
The Update operation is quite similar to the create operation. First, let’s build UI by creating a new custom column on the table by clicking on ADD A NEW COLUMN under the columns property.
Now, rename the column to Edit, and click on the cog icon next to it, to configure column settings. Under this, we’ll see column-type properties set to a Button type. When clicked, a modal should open up with the necessary fields to update the item.
Now, copy-paste Modal1 and rename it to Modal2, and set the onClick property of the Edit Task button to open Modal2. Here, in the form, we can also set the default value to show existing information, to display this, use the selectedRow property from the table widget.
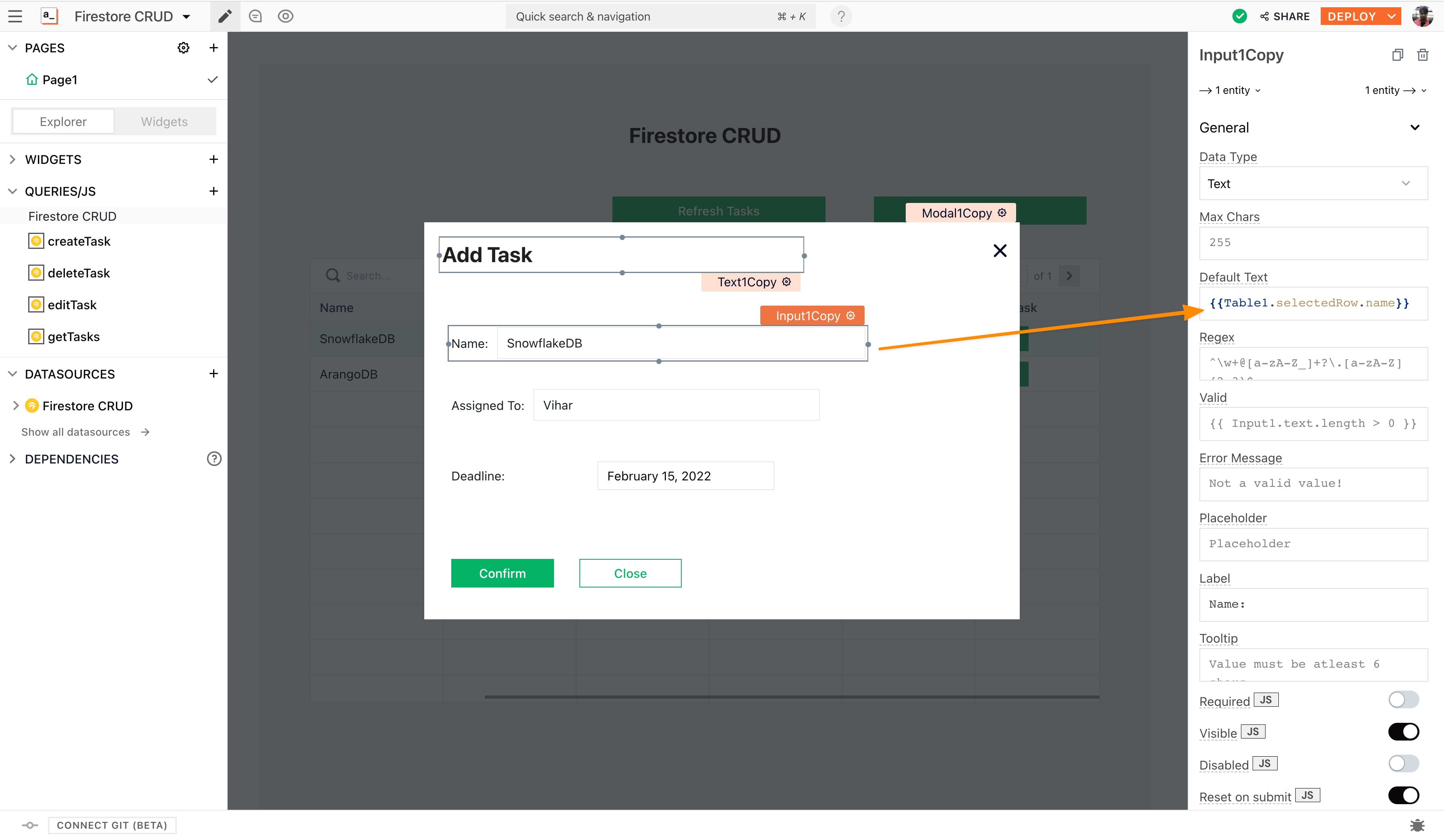
Let’s write the Edit query:
Click on the +icon next to the datasources and choose to Create New + from the Firestore CRUD datasource
Rename the query to editTask
Set the Collection/Document Path* to tasks/{{Table1.selectedRow._ref}}
Finally, set the body property to:
{
"name":"{{Input1Copy.text}}",
"deadline": "{{DatePicker1Copy.formattedDate}}",
"assigned_to": "{{Input2Copy.text}}",
"complete": false
}
“”Note: The {{ Table1.selectedRow._ref }} snippet evaluates to the selected row’s _ref which will be the row we want to edit to.
Here, we have an edit query that collects all the data from the form widgets on Modal2. Note that we use the moustache syntax to bind the data from the widgets onto the query body.
We’ll now need to configure the submit button; for this, go back to Modal2 and set the button’s onClick property to execute a query and choose **_editTask_** under the events property.
Implementing the Delete Operation
The delete operation is pretty straightforward with the Table’s selectedRow property; before we dive into it, let’s create a new column on the table and set it to the button. For this:
Create a new custom column on the table by clicking on
Add a New Column
under the columns property.
Now, rename this column to ‘Delete Task,’ and click on the cog icon next to it, to configure column settings. Under this, we’ll see column-type properties set to a button type.
Now, let’s write the Delete query:
Click on the + icon next to the data sources and choose the Create New + from the Firestore CRUD datasource
Set the commands to Delete Document
Set the Collection/Document Path* toSet the Delete Task button’s onClick property to run the deleteTask query.
tasks/{{Table1.selectedRow._ref}}
With these four operations configured, you will be able to read and analyze information from your database, edit the data, add or delete information and update records.
If you’re interested in using a database that is not listed on our website as an integration, please let us know about it by raising a PR on Github and we will do our best to include it at the earliest.
Join our growing community on Discord, and follow us on Youtube and Twitter to stay up to date.
Related Blog Posts
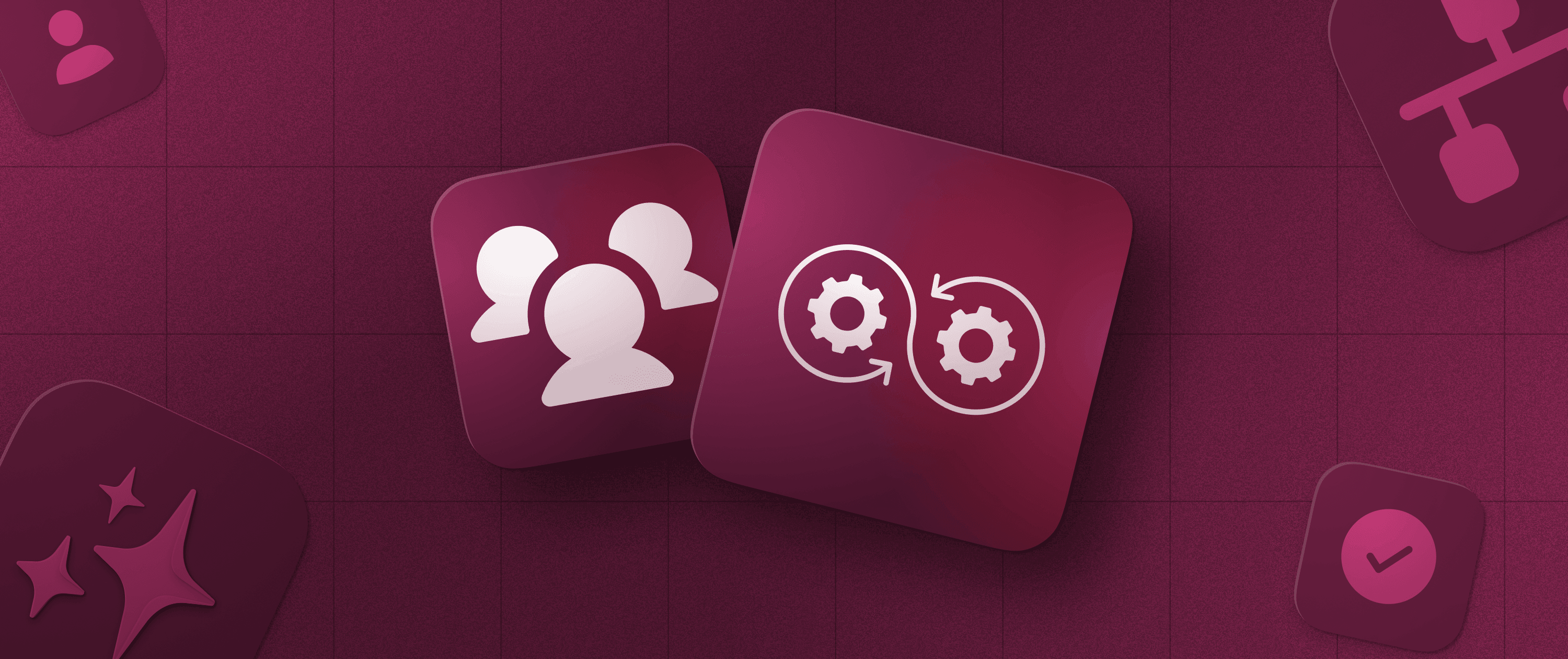

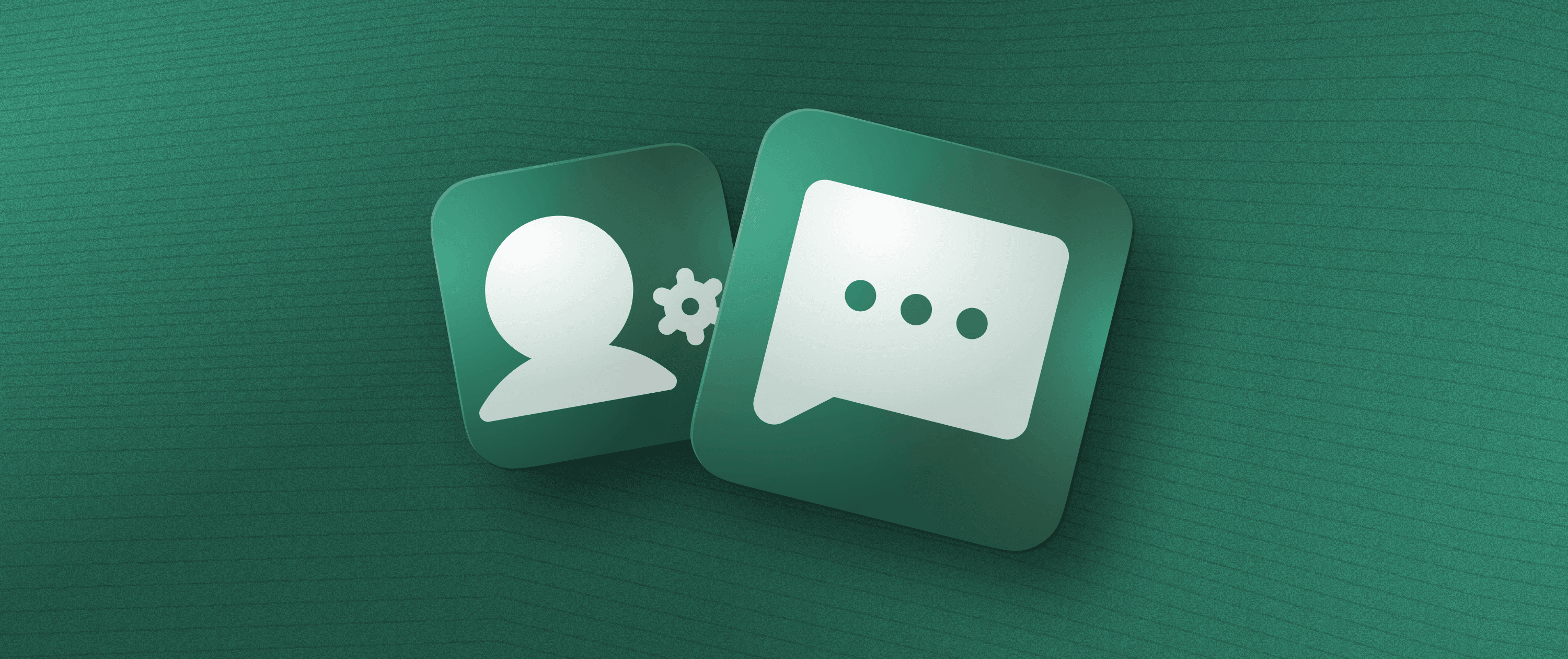
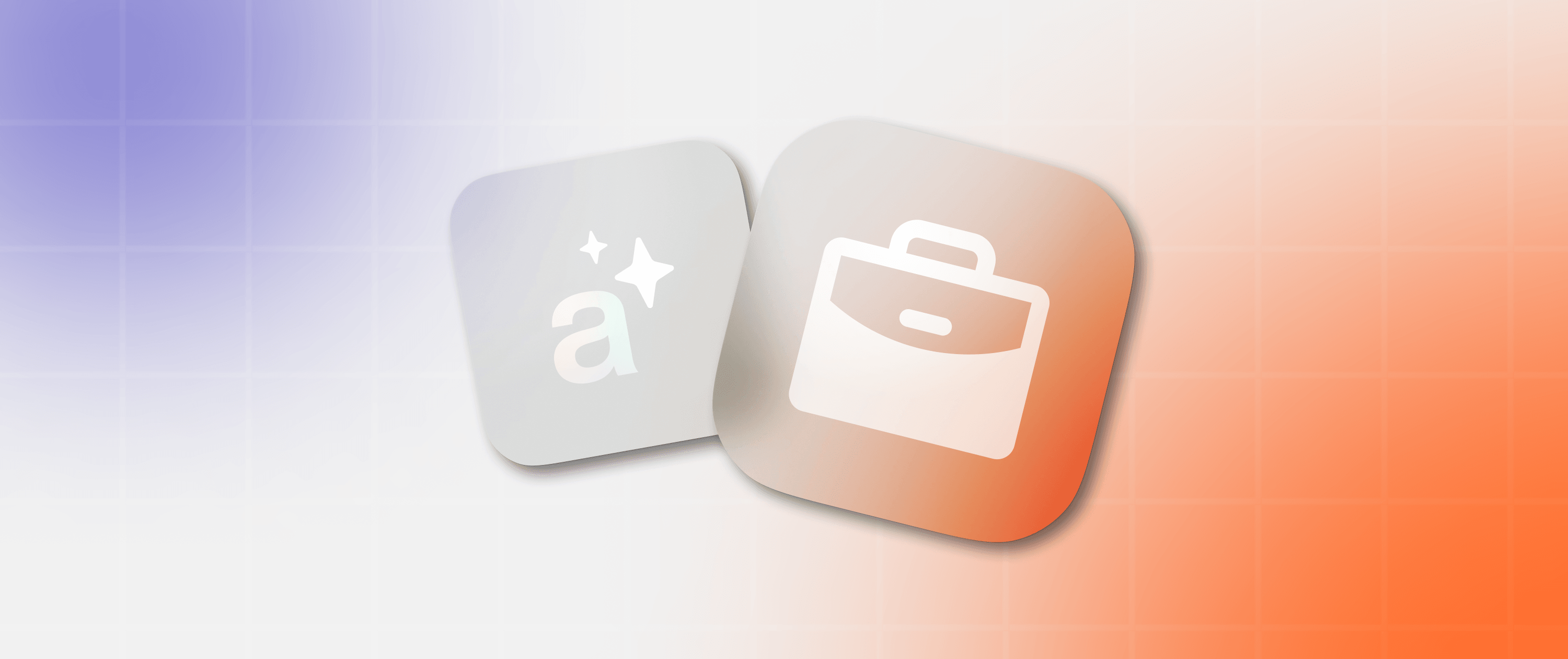
